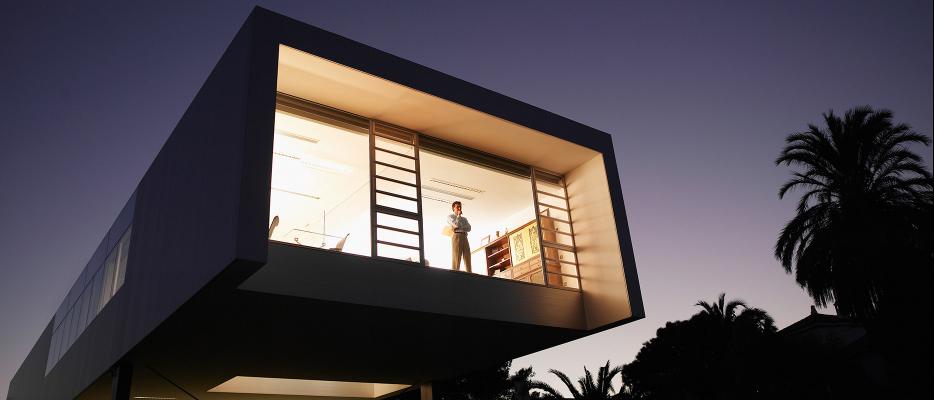
There are a few basic things a developer needs to do in a custom module.... SELECT, INSERT and DELETE queries are top of my list and this article demonstrates a few examples of SELECT and INSERT queries.
This code is within a controller that is part of a custom module. I won't explain how to build the module but this code is within a file called ReportController.php.
The basic file code is:
namespace Drupal\mycustommodule\Controller; use Drupal\Core\Controller\ControllerBase; use Drupal\Core\Database\Database; class ReportController extends ControllerBase { // The main controller code goes in here }
I put my queries into separate 'protected' functions which are called within the main 'public' function like this:
public function mainReportFunction() { $savedVideos = $this->getVideoIds(); }
I won't explain how the following functions work but they're basic SQL SELECT with a foreach() to return clean values or arrays.
// SELECT protected function getVideoIds() { $select = Database::getConnection()->select('your_videos', 'yv'); $select->addField('yv', 'yt_id'); $entries = $select->execute()->fetchAll(\PDO::FETCH_ASSOC); $savedVideos = []; foreach ($entries as $v) { $savedVideos[$v['yt_id']] = true; } return $savedVideos; }
// SELECT protected function getNodeIdUsingVideoId($savedVideoId) { $select = Database::getConnection()->select('node__field_video_id', 'nfvid'); $select->condition('nfvid.field_video_id_value', $savedVideoId, '='); $select->fields('nfvid', ['entity_id']); $select->range(0, 1); $entries = $select->execute()->fetchAll(\PDO::FETCH_ASSOC); if(isset($entries[0]['entity_id'])){ return $entries[0]['entity_id']; } return false; }
// INSERT protected function addPlaylistEntry($ply) { $connection = \Drupal::service('database'); $result = $connection->insert('video_playlist') ->fields([ 'title' => $ply['name'] , 'playlist_id' => $ply['id'] , 'description' => $ply['description'] , 'thumbnail_high' => $ply['thumbnail_url'] ]) ->execute(); if(!is_numeric($result)){ \Drupal::logger('mycustommodule')->error('Error INSERT failed: '.$ply['id']); } return $result; // result is the row id }
// DELETE protected function deleteVideoById($vidId) { $delete = Database::getConnection()->delete('videos_table'); $delete->condition('video_id', $vidId); $delete->execute(); return true; }
Related Articles
Main Category
Secondary Categories