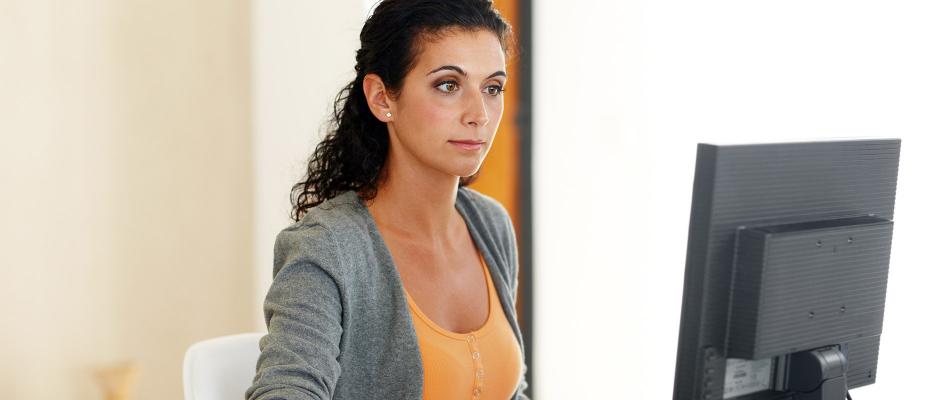
There are a few basic things a developer needs to do in a custom module.... node creation is top of my list.
This code is within a controller that is part of a custom module. I won't explain how to build the module but this code is within a file called ReportController.php.
The basic file code is:
namespace Drupal\mycustommodule\Controller; use Drupal\Core\Controller\ControllerBase; use Drupal\node\Entity\Node; class ReportController extends ControllerBase { // The main controller code goes in here }
I put the node creation code into a separate 'protected' function which are called within the main 'public' function like this:
public function mainReportFunction() { $newVideoNode = $this->createVideoNode($video); }
I won't explain how the following functions work but they're basic SQL SELECT with a foreach() to return clean values or arrays.
protected function createVideoNode($vid) { $newVideo = Node::create(['type' => 'video']); $newVideo->set('title', $vid['title']); $newVideo->set('body', $vid['description']); $newVideo->set('field_video_id', $vid['id']); $newVideo->set('field_thumbnail', $vid['thumbnail_url']); $newVideo->set('field_published_at', $vid['created_time']); $newVideo->enforceIsNew(); $newVideo->save(); return true; }
Related Articles
Main Category
Secondary Categories