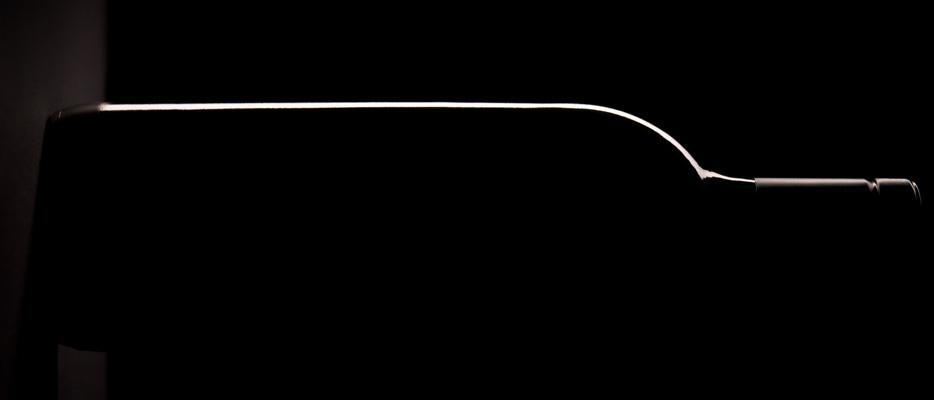
Email validation is something that is needed in a variety of situations, but I’ve always struggled to find a good one…. Well, here are 2!
The first is something I found online somewhere, and has been modified a little…
$email = '[email protected]'; if(preg_match("/^[_a-z0-9-]+(\.[_a-z0-9-]+)*@[a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3})+$/i", $email)) { // All OK, do something } else { // Not OK, throw error }
The second is from the legendary Mark Jackson, it requires PHP5 and in his words:
PHP actually has a built in function called filter_var which has an email validation flag in it but it will allow for any hostname (like mark@localhost) which I do not like so I have added another simple check to make sure it has a dot after the @ sign the function I use is:
function checkEmail($e,$bypassVal=false) { // ensure the email has a valid extension if(($bypassVal!==false)&&(is_array($bypassVal))&&(in_array($e,$bypassVal))) { return true; } if(strpos($e,'@')>=strrpos($e,'.')) { return false; } return (filter_var($e, FILTER_VALIDATE_EMAIL) && preg_match('/@.+\./', $e)) ? true : false; }
The $bypassVal variable allows you to specify any email addresses (in an array format) which can can go through without validation – just in case you want to specify a particular string or email which can get through.
Examples
checkEmail(‘[email protected]’); // returns true
checkEmail(‘mark@localhost’); // returns false
checkEmail(‘mark@localhost’,array(‘mark@localhost’)); // returns true
checkEmail(‘__I_GET_THROUGH__’,array(‘mark@localhost’,'__I_GET_THROUGH__’)); // returns true