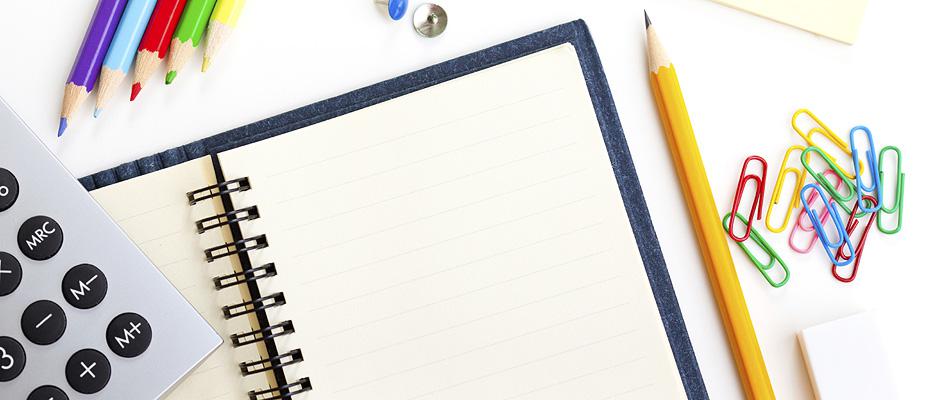
This is the most recent version of the script I’m using… Copy and Paste the code straight into a page and save it as yourfilename.php, and that’s about it…. You just need to change the name and e-mail address from mine to yours…
<?php session_start(); // Start Sessions for calculation // Functions and Variables, etc. Ignore unless you want to change the email's priority level, (Line 20). // checkStr() and noNewLines() look for abuse of the form. // purify() removes html characters, which will also remove <script> tags white space and add new lines. // topNtail() can be expanded to add html headers and footers too. // $headers variable sets bits and bobs fro the html email. function checkStr($str) { $strings = array("content-type:","mime-version:","multipart/mixed","Content-Transfer-Encoding:","bcc:","cc:","to:"); foreach($strings as $string) { if(eregi($string, strtolower($str))) { return true; } } } function noNewLines($str) { if (eregi("(\r|\n)", $str)) { return true; } } function purify($text) { return nl2br(htmlspecialchars(trim($text), ENT_QUOTES, 'UTF-8')); } function topNtail($message) { return '<html><body>'.$message.'</body></html>'; } $headers = 'MIME-Version: 1.0' . "\r\n"; $headers .= 'Content-type: text/html; charset="iso-8859-1"' . "\r\n"; $headers .= 'X-Priority: 3' . "\r\n"; // change to 1 for top priority, 3 is normal, 5 is low. $headers .= 'X-Mailer: PHP/' . phpversion(); /* ##################################################################################################### */ /* ###################### ############################ */ /* ###################### Variables & Settings for the PHP Contact Form ############################ */ /* ###################### ############################ */ /* ##################################################################################################### */ // Path to the "thanks for the message" page. Customers get redirected here once the e=mail has been sent. $thankyoupage = 'index.php'; // thankyou.html // Set to 'true' if you want the person who filled the form in to receive a confirmation message. $sendnotification = true; // Set the page for the form to be submitted to. Leave blank unless you need to split it, i,e, Ajax. $contact_form_action = ""; // /* ##################################################################################################### */ /* #################### ########################## */ /* #################### Settings for the e-mail to you from your customer ########################## */ /* #################### ########################## */ /* ##################################################################################################### */ // Your details $youremail = '[email protected]'; $yourname = 'Your Name'; // First line of e-mail to self. $contact_name = purify($_POST['contact_name']); // This line is needed to set the next 2 variables $contact_comment = "Here is the message $contact_name sent from the form on your contact page"."\n"."\n"; // Appears in the subject field of the e-mail send to yourself. $subjectline = "$contact_name has sent you a message from your website."; /* ##################################################################################################### */ /* ########################## ################################# */ /* ########################## Settings for e-mail to your customer ################################# */ /* ########################## ################################# */ /* ##################################################################################################### */ // This is the confirmation message that will be sent to your customer. $notification_message = "<p>Thank you for your e-mail. I will be in-touch shortly</p>" ."\n"."\n". "<p>If you need to contact me quickly, please use one of the following</p>" ."\n"."\n". "<p>T: 01234 567890<br />" ."\n". "M: 01234 567890<br />" ."\n". "E: <a href=\"mailto:$youremail\">$youremail</a></p>" ."\n"."\n". "<p>Kindest Regards<br />" ."\n". "$yourname</p>"; // This is appears in the subject line of the confirmation message that will be sent to your customer. $notification_subject = "Thank you for contacting $yourname"; /* ##################################################################################################### */ /* ####################################### ########################################### */ /* ####################################### Main Workings ########################################### */ /* ####################################### ########################################### */ /* ##################################################################################################### */ if ((isset($_POST["fromContactForm"])) && ($_POST["fromContactForm"] == "bingo")) { // Create a form error array for every field you completed in order for the form to be allowed to be submitted. // For example, make sure you have the main name and e-mail address listed below. $err = 0; $contacter_form_error = array(); if (empty($_POST['answer'])) { $contacter_form_error[] = 'the validation equation'; $err++; } if (empty($_POST['contact_name'])) { $contacter_form_error[] = 'your name'; $err++; } if (empty($_POST['contact_email'])) { $contacter_form_error[] = 'your email address'; $err++; } if (empty($_POST['how_did_you_hear'])) { $contacter_form_error[] = 'how you heard about us'; $err++; } if($err == 0) { // Check for abuse if(noNewLines($_POST['contact_email']) == true || noNewLines($_POST['contact_name']) == true || checkStr($_POST['message1']) == true) { $errmsg == TRUE; } else { // If all fields and validation equation were filled in... check equation... $answer = trim($_POST['answer']); if($answer != $_SESSION['answer']) { $answerError = TRUE; } else { $contact_name = purify($_POST['contact_name']); $contact_email = purify($_POST['contact_email']); $contact_comment .= '<p><strong>Name:</strong> '.$contact_name."</p> \n"; $contact_comment .= '<p><strong>Email:</strong> '.$contact_email."</p> \n"; if(!empty($_POST['message1'])){ $contact_comment .= '<p><strong>Message:</strong><br />'.purify($_POST['message1'])."</p> \n"; } if(!empty($_POST['contact_phone'])){ $contact_comment .= '<p><strong>Tel:</strong> '.purify($_POST['contact_phone'])."</p> \n"; } if(!empty($_POST['contact_phone2'])){ $contact_comment .= '<p><strong>Mob:</strong> '.purify($_POST['contact_phone2'])."</p> \n"; } if(!empty($_POST['how_did_you_hear'])){ $contact_comment .= '<p><strong>How did you hear about us:</strong><br />'.purify($_POST['how_did_you_hear'])."</p> \n"; } // Send e-mail to yourself from the customer who filled the form in. mail($youremail, $subjectline, topNtail($contact_comment), "From: $contact_email\r\n".$headers); // Send an e-mail to your customer. if($sendnotification == true){ mail($contact_email, $notification_subject, topNtail($notification_message), "From: $youremail\r\n".$headers); } // Once complete. Redirect to the thank you page. header("Location:$thankyoupage"); exit(); } // end else answer } // end else abuse } // end if $err } // Close of 'if ((isset($_POST["fromContactForm"]))' brackets... ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Contact Page</title> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <style type="text/css"> <!-- .highlight-text { color: #900; } --> </style> </head> <body> <h2>Contact Me</h2> <p>The easiest way to contact me is via the form below.</p> <p>If you need to get a hold of me immediately, please complete the form below and my contact numbers will be e-mailed to you within a few minutes.</p> <?php // Print form field errors if present if (count($contacter_form_error)>0){ echo "<p>Please insert "; foreach($contacter_form_error as $form_err){ if ($err > 2) { echo "<span class=\"highlight-text\">$form_err</span>, "; $err--; } elseif ($err > 1) { echo "<span class=\"highlight-text\">$form_err</span> and "; $err--; } else { echo "<span class=\"highlight-text\">$form_err</span>."; } } echo "</span></p>"; } // Check to see if answer error is true. if($answerError == TRUE) { echo "<p><span class=\"highlight-text\">Your answer was wrong! Please try again.</span>.</span></p>"; } ?> <form action="<? print $contact_form_action; ?>" method="post"> <table cellpadding="2" cellspacing="0" id="contact"> <tr> <td><label for="contact_name">Your Full Name</label>:<span>*</span></td> <td><input type="text" id="contact_name" name="contact_name" size="40" value="<? print $_POST['contact_name']; ?>" /></td> </tr> <tr> <td><label for="contact_email">Your Email Address</label>:<span>*</span></td> <td><input type="text" id="contact_email" name="contact_email" size="40" value="<? print $_POST['contact_email']; ?>" /></td> </tr> <tr> <td><label for="contact_phone">Telephone No.</label>:</td> <td><input type="text" id="contact_phone" name="contact_phone" size="25" value="<? print $_POST['contact_phone']; ?>" /> (Day Time, Work or Home) </td> </tr> <tr> <td><label for="contact_phone2">Mobile No.</label>:</td> <td><input type="text" id="contact_phone2" name="contact_phone2" size="25" value="<? print $_POST['contact_phone2']; ?>" /> (Secondary Contact Number) </td> </tr> <tr> <td><label for="message1">Message</label>:</td> <td><textarea name="message1" cols="55" rows="7" id="message1"><? print $_POST['message1']; ?></textarea></td> </tr> <tr> <td><label for="how_did_you_hear">How did you hear about us?</label><span>*</span></td> <td><input type="text" id="how_did_you_hear" name="how_did_you_hear" size="40" value="<? print $_POST['how_did_you_hear']; ?>" /></td> </tr> <tr><td colspan="2"> </td></tr> <tr> <td colspan="2"> For Security Reasons : In order to avoid my contact form from being abused <br /> by computer generated programs, please solve the following equation. </td> </tr> <tr> <td><label for="answer">Validation equation</label>:<span>*</span></td> <td> <?php // generate 2 random numbers for the validation equation... and write to Session.. $randomNumber1 = rand(1,10); $randomNumber2 = rand(1,10); $_SESSION['answer'] = $randomNumber1 + $randomNumber2; echo $randomNumber1 . ' + ' . $randomNumber2 . ' = '; ?> <input name="answer" type="text" id="answer" size="2" /> </td> </tr> <tr><td colspan="2"> </td></tr> <tr> <td> </td> <td> <input type="hidden" name="fromContactForm" value="bingo" /> <input type="submit" value="Send Mesage" /> </td> </tr> <tr> <td colspan="2"><p><span>* Please make sure these fields have been filled in.</span> </p></td> </tr> </table> </form> </body> </html>
Main Category