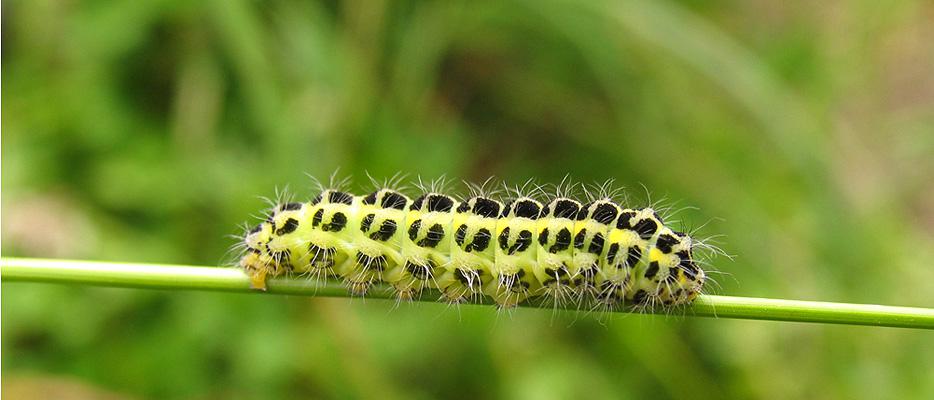
I’ve become a big fan of using a ‘config’ file that I include at the beginning of all my PHP pages. Here I can set everything from global settings and variables, to site-wide functions…
One of the site-wide functions I use a lot sends an email if a script fails, i.e. do this and if it worked do this, else email error… It’s handy with database INSERT and UPDATE as you can let your user carry on without seeing an error, and then email yourself the entire SQL query for analysis and manual entry….
In my config.php file I have:
// // Function to handle errors (DB) // function emailError($message){ $toEmail = '[email protected]'; $fromEmail = '[email protected]'; $subjectline = 'Error from Site Name'; // send e-mail mail($toEmail, $subjectline, $message, "From: fromEmail"); }
To use just trigger like…
emailError('Error: What was supposed to happen has failed!!!');
Or with a database function…
$query = 'INSERT INTO `table` (`col1`,`col2`,`timestamp`) VALUES ("'.$val1.'", "'.$val2.'", NOW())'; $result = @mysql_query($query); if(mysql_affected_rows()==0){ emailError('Error: Failed to add entry to DB'."\n" .'Query: '.$query."\n" .'URL: '.$_SERVER["REQUEST_URI"]."\n" .'MySQL Error: '.mysql_error()."\n"); }
Update
I’ve developed this a little further to include the creation or update of a log file… Plus the added ability to NOT send an email, so you can catch ‘events’ as well as ‘errors’ in the log file without bombarding yourself with lots of emails. I’ve also used DEFINE but you can use normal variables if you prefer.
Here is how the function looks now…
// Set variables or constants define('ERROR_LOG_PATH',$_SERVER['DOCUMENT_ROOT'].'/path-to-folder/'); // set error log path define('ERROR_LOG_NAME','error-log.txt'); // set error log file name define('ADMIN_EMAIL','[email protected]'); // set who to email define('ERROR_SUBJECT','Error from Site Name'); // set email subject define('SITE_EMAIL','[email protected]'); // set who sent email function emailError($message,$email=true){ // 1st write error to log file $f = fopen (ERROR_LOG_PATH.ERROR_LOG_NAME,'a'); fputs($f, date('d/m/Y H:i:s').' - '.$message."\n"); // write date, hyphen, message on a new line. fclose($f); // 2nd email administrator if($email==true){ mail(ADMIN_EMAIL, ERROR_SUBJECT, mysql_real_escape_string($message), "From: ".SITE_EMAIL); // send e-mail } } // end function
You still call it the same way, but you’ll notice in the function parameters (emailError($message,$email=true)) that $email is set to true by default. This means that an email will be sent if just the message is passed, but you can also add false if you just want to log the ‘event’…. i.e.
emailError(‘Something failed!!!’); will log and send an email.
emailError(‘Something happened’, false); will log the message, but not send an email.
This script produces something like…
23/11/2011 11:32:02 - Database update success - company - id:576 25/11/2011 17:14:08 - User login failed, wrong password, un: Bob. 25/11/2011 17:14:42 - User login success, un: Bob.
If the file is not being created, check the folder permissions… chances are, the ‘write’ permissions are wrong…. Caught me out a few times!!!