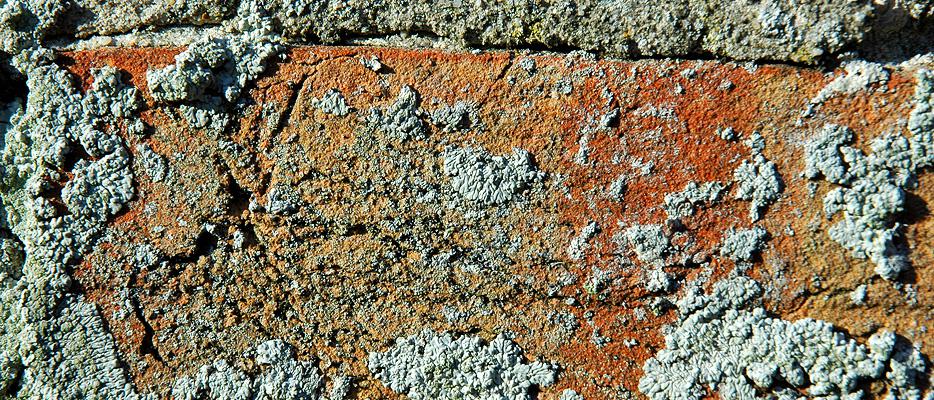
Here’s another way to connect to the database:
// Connect with username, password, etc... $link = mysqli_connect('localhost', 'userName', 'passWord'); if (!$link){ $error = 'Unable to connect to the database server.'; include 'error.html.php'; exit(); } // Set Charset if (!mysqli_set_charset($link, 'utf8')){ $error = 'Unable to set database connection encoding.'; include 'error.html.php'; exit(); } // select database if (!mysqli_select_db($link, 'ijdb')){ $error = 'Unable to locate the database.'; include 'error.html.php'; exit(); }
This script runs through the different tasks, and if it’s successful, it moves onto the next. If there is a problem, a message is saved to the $error variable, and a HTML template is included to show it.
Writing queries is slightly different, and goes like this:
$result = mysqli_query($link, 'SELECT * FROM tableName WHERE conditions'); if (!$result){ $error = 'Error with query!'; include 'error.html.php'; exit(); } while ($row = mysqli_fetch_array($result)){ var1 = $row['col1']; var2 = $row['col2']; var3 = $row['col3']; // etc... }
Main Category
Secondary Categories