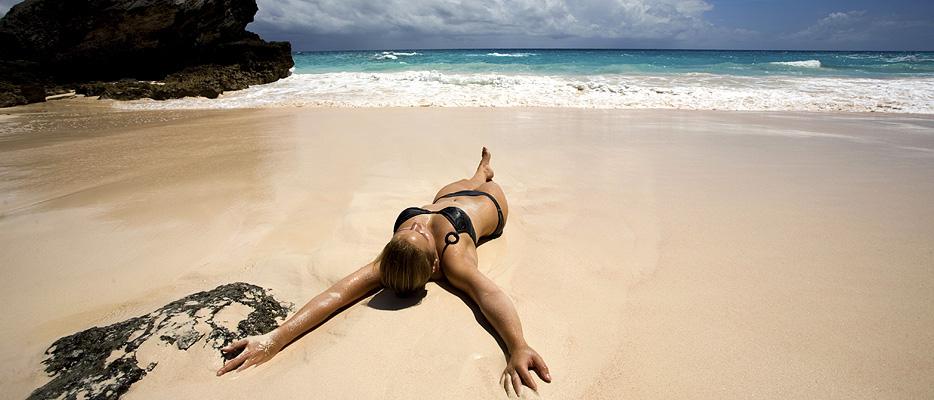
Quick Reference guide of dot operators:
.length // counts the number of items in an array
.nodeName // reference the name of the node, i.e. p for p tag, etc.
.parentNode // references the parent on an element node
.childNodes // references the elements of children. i.e. the parent can be a <ul> tag and the children would be the <li> tags. You reference them as you arrays, .childNodes[0] onwards.
.firstChild // as above, but just gets the 1st child... same as .childNodes[0]
.lastChild // as above, but gets the last child... same as childNodes[childNodes.length - 1]
.nextSibling // if using the <ul><li> example, and you referenced the <li> tag, this will get the next <li> tag in the list.
.previousSibling // as above but the other way round.
.getAttribute() // returns the attribute in the brackets, i.e. myTitle = myVar.getAttribute("title");
.href // you can just reference the attribute with the dot operator as you would an object. i.e. myTitle = myVar.href;
.setAttribute("attribute", "value") // i.e. myVar.setAttribute("href", "/koko/");
.style // followed by the CSS property, can change the CSS.
.style.color // i.e. myElement.style.color = "#FF0000";
.className // myElement.className = "my-css-class";
.createElement() // creating new elements to add to the html, i.e. document.createElement("span");
.createTextNode() // creating new content text to add to an element, i.e. document.createTextNode("Text goes here");
.appendChild() // adding a child element to an existing node, i.e. tip.appendChild(tipText);
.nextSibling
.nodeValue
.removeChild() // i.e. removeChild(tip)
Main Category