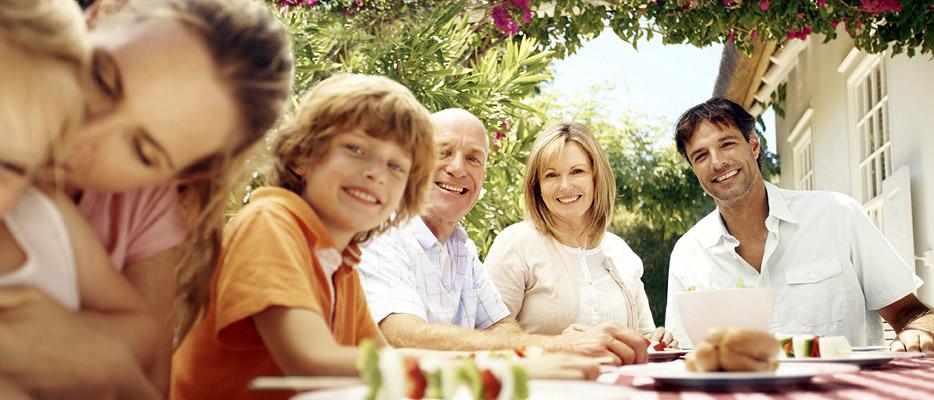
I often want to pull something out onto a page, from the node, but into a region not covered in node.tpl.php... Lets say for example, a page has a 'banner' sat below the header and under the banner is another region, and below that is the normal content area... We can use the page.tpl.php, check if $node exists (rather than a system page), even check the $node->type, and then render the image.
For example, lets say I have a content type called 'sponsor', and for this content type I've created a field called 'Single Banner Image' that allows the admin team to upload a single image that overwrites the slider that appears by default. I can get the array using $node->field_single_banner_image and then the actual 'uri' using $node->field_single_banner_image['und'][0]['uri'] however, it's a bit clumsy and it doesn't handle any of the other fields, alt tags, title tags, etc... So how do we tackle this the 'correct' way?
The first step is to drop an if statement in to catch the node type, so in my case, the slider runs on all pages except those that are a 'sponsor' content type. This can be set by the slider block, but the if statement could be doing other things, so just follow my thinking for a moment....
<?php if(isset($node->type) && $node->type == 'sponsor'){ // show page loaded banner for sponsor pages ?> <p>HTML goes in here for the sponsor banner</p> <?php } else { ?> <p>Default slider goes in here</p> <?php } // end else ?>
Inside the top part of the if statement, we can put something like:
<div class="container"> <?php if(isset($node->field_single_banner_image)){ $field = field_get_items('node', $node, 'field_single_banner_image'); $bannerImage = field_view_value('node', $node, 'field_single_banner_image', $field[0]); print render($bannerImage); } ?> </div>
... that's it...
field_view_value() can also take an extra parameter at the end, which can be used for various setting... Lets say we want to use the 'thumbnail' image style (or any other image style you have created, just make sure you use the 'machine name'), and link it to the content (node the image was uploaded to)... We can create an array called $options like:
$bannerOptions = array( 'type' => 'image' , 'settings' => array( 'image_style' => 'thumbnail' , 'image_link' => 'content' ) );
So the whole thing together with the $options is:
<div class="container"> <?php if(isset($node->field_single_banner_image)){ $field = field_get_items('node', $node, 'field_single_banner_image'); $bannerOptions = array( 'type' => 'image' , 'settings' => array( 'image_style' => 'thumbnail' , 'image_link' => 'content' ) ); $bannerImage = field_view_value('node', $node, 'field_single_banner_image', $field[0], $bannerOptions); print render($bannerImage); } ?> </div>